Angular7 Materials/CDK-虚拟滚动
这是 Angular 7 添加的新功能之一,称为虚拟滚动。此功能已添加到 CDK(组件开发工具包)中。虚拟滚动向用户显示可见的 dom 元素,当用户滚动时,会显示下一个列表。这提供了更快的体验,因为完整列表不会一次性加载,而只会根据屏幕上的可见性加载。
为什么我们需要虚拟滚动模块?
考虑你有一个 UI 有一个很大的列表,在其中加载所有数据可能会出现性能问题。 Angular 7 虚拟滚动的新特性负责加载用户可见的元素。当用户滚动时,将显示下一个用户可见的 dom 元素列表。这提供了更快的体验,并且滚动也非常流畅。
让我们将依赖添加到我们的项目中:
npm install @angular/cdk –save

我们已完成安装虚拟滚动模块的依赖项。
我们将通过一个示例来更好地理解如何在项目中使用虚拟滚动模块。
我们将首先在里面添加虚拟滚动模块 app.module.ts 如下:
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule , RoutingComponent} from './app-routing.module'; import { AppComponent } from './app.component'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { ChangeTextDirective } from './change-text.directive'; import { SqrtPipe } from './app.sqrt'; import { MyserviceService } from './myservice.service'; import { HttpClientModule } from '@angular/common/http'; import { ScrollDispatchModule } from '@angular/cdk/scrolling'; @NgModule({ declarations: [ SqrtPipe, AppComponent, NewCmpComponent, ChangeTextDirective, RoutingComponent ], imports: [ BrowserModule, AppRoutingModule, HttpClientModule, ScrollDispatchModule ], providers: [MyserviceService], bootstrap: [AppComponent] }) export class AppModule { }
在 app.module.ts 中,我们导入了 ScrollDispatchModule 并将其添加到导入数组中,如上面的代码所示。
下一步是获取要在屏幕上显示的数据。我们将继续使用我们在上一章创建的服务。
我们将从 url 获取数据, https://jsonplaceholder.typicode.com/photos 其中包含大约 5000 张图像的数据。我们将从中获取数据并使用虚拟滚动模块显示给用户。
网址中的详细信息, https://jsonplaceholder.typicode.com/photos 如下面所述:
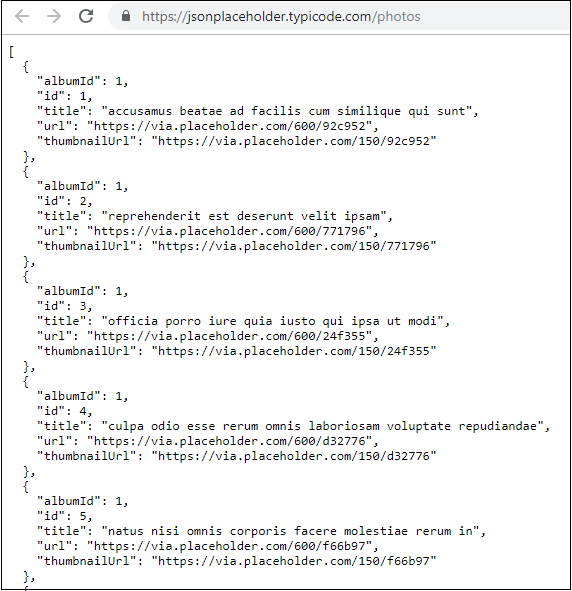
它是具有图像 url 和缩略图 url 的 json 数据。我们将向用户显示缩略图 url。
以下是获取数据的服务:
我的服务.service.ts
import { Injectable } from '@angular/core'; import { HttpClient } from '@angular/common/http'; @Injectable({ providedIn: 'root' }) export class MyserviceService { private finaldata = []; private apiurl = "https:// jsonplaceholder.typicode.com/photos"; constructor(private http: HttpClient) { } getData() { return this.http.get(this.apiurl); } }
我们将从 app.component.ts 调用服务如下:
import { Component } from '@angular/core'; import { MyserviceService } from './myservice.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7 Project!'; public albumdetails = []; constructor(private myservice: MyserviceService) {} ngOnInit() { this.myservice.getData().subscribe((data) => { this.albumdetails = Array.from(Object.keys(data), k=>data[k]); console.log(this.albumdetails); }); } }
现在变量 专辑详情 拥有来自 api 的所有数据,总数为 5000。
现在我们已经准备好显示数据,让我们在 app.component.html 中工作以显示数据。
我们需要添加标签,
这是
<h3>Angular 7 - Virtual Scrolling</h3> <cdk-virtual-scroll-viewport [itemSize] = "20"> <table> <thead> <tr> <td>ID</td> <td>ThumbNail</td> </tr> </thead> <tbody> <tr *cdkVirtualFor = "let album of albumdetails"> <td>{{album.id}}</td> <td> <img src = "{{album.thumbnailUrl}}" width = "100" height = "100"/> </td> </tr> </tbody> </table> </cdk-virtual-scroll-viewport>
我们在屏幕上向用户显示 id 和缩略图 url。到目前为止,我们主要使用 *ngFor,但在内部
我们正在遍历 app.component.html 中填充的 albumdetails 变量。为虚拟标签 [itemSize]="20" 分配了一个大小,它将根据虚拟滚动模块的高度显示项目的数量。
虚拟滚动模块相关的css如下:
table { width: 100%; } cdk-virtual-scroll-viewport { height: 500px; }
赋予虚拟滚动的高度为 500px。适合该高度的图像将显示给用户。我们完成了添加必要的代码来查看我们的虚拟滚动模块。
Virtual Scroll Module 在浏览器中的输出如下:
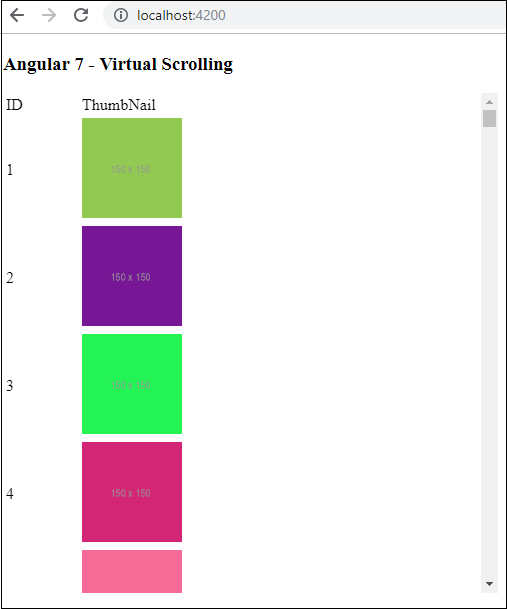
我们可以看到前 4 张图像显示给用户。我们已经指定了 500px 的高度。表格有滚动显示,当用户滚动时,将显示适合该高度的图像,如下所示:
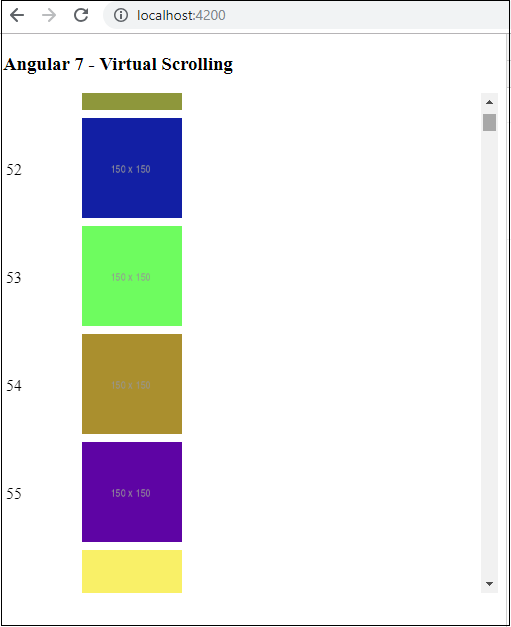
用户滚动时会加载所需的图像。此功能在性能方面非常有用。起初,它不会加载所有 5000 张图像,而是在用户滚动时调用并显示 url。