Angular7 数据绑定
数据绑定可直接从 AngularJS 获得,并且所有版本的 Angular 都在稍后发布。我们使用花括号进行数据绑定 - {{}};这个过程称为插值。我们已经在前面的示例中看到了如何将值声明给变量 title 并在浏览器中打印相同的值。
中的变量 app.component.html 文件被称为 {{标题}} 和价值 title 在初始化 app.component.ts 文件并在 app.component.html ,显示值。
现在让我们在浏览器中创建月份的下拉列表。为此,我们在 app.component.ts 如下:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // 声明的月份数组。 months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; }
上面显示的月份数组将显示在浏览器的下拉列表中。
我们已经创建了带有选项的普通选择标签。在选项中,我们使用了 for loop . The for loop 用于遍历月份的数组,这反过来将创建具有月份中存在的值的选项标签。
Angular中的语法如下:
*ngFor = “let I of months”
并获得月份的值,我们将其显示在:
{{i}}
两个大括号有助于数据绑定。你在 app.component.ts 文件中声明变量,并且将使用大括号替换相同的变量。
以下是上述月份数组在浏览器中的输出:

中设置的变量 app.component.ts 可以绑定在里面 app.component.html 使用大括号。例如: {{}}。
现在让我们根据条件在浏览器中显示数据。在这里,我们添加了一个变量并将其赋值为 true .使用 if 语句,我们可以隐藏/显示要显示的内容。
例子
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // 声明的月份数组。 months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = true; // 变量设置为真 }
app.component.html
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1> Welcome to {{title}}. </h1> </div> <div> Months : <select> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable">Condition is valid.</span> // 这里根据 if 条件显示文本条件有效。 // 如果 isavailable 的值设置为 false 则不会显示文本。 </div>
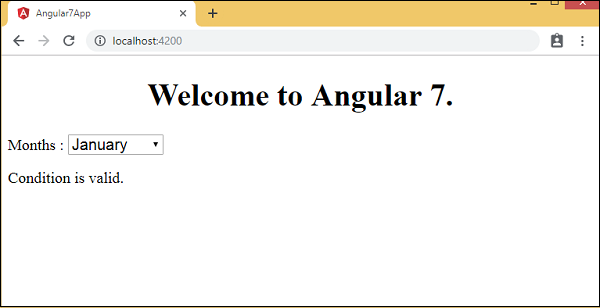
让我们使用 否则 健康)状况。
例子
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // 声明的月份数组。 months = ["January", "Feburary", "March", "April", "May","June", "July", "August", "September", "October", "November", "December"]; isavailable = false; // 变量设置为真 }
在这种情况下,我们制作了 可用 变量为假。要打印 else 条件下,我们将不得不创建 ng-模板 如下:
<ng-template #condition1>Condition is invalid</ng-template>
完整代码如下:
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1> Welcome to {{title}}. </h1> </div> <div> Months : <select> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable; else condition1">Condition is valid.</span> <ng-template #condition1>Condition is invalid</ng-template> </div>
If 与 else 条件一起使用,使用的变量是 条件1 .同样被分配为 id to the ng-模板 ,并且当可用变量设置为 false 时,文本 条件无效 被展示。
以下截图显示在浏览器中的显示:

现在让我们使用 如果 那么 否则 健康)状况。
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 7'; // 声明的月份数组。 months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = true; // 变量设置为真 }
现在,我们将创建变量 可用 作为真实。在html中,条件是这样写的:
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1> Welcome to {{title}}. </h1> </div> <div> Months : <select> <option *ngFor="let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable; then condition1 else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid</ng-template> <ng-template #condition2>Condition is invalid</ng-template> </div>
如果变量为真,那么 条件1 , else 条件2 .现在,使用 id 创建了两个模板 #条件1 and #条件2 .
在浏览器中显示如下:
