VueJS Mixins
Mixins基本上与组件一起使用。它们在组件之间共享可重用的代码。当组件使用mixin时,mixin的所有选项都将成为组件选项的一部分。
Example
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"></div> <script type = "text/javascript"> var vm = new Vue({ el: '#databinding', data: { }, methods : { }, }); var myMixin = { created: function () { this.startmixin() }, methods: { startmixin: function () { alert("Welcome to mixin example"); } } }; var Component = Vue.extend({ mixins: [myMixin] }) var component = new Component(); </script> </body> </html>
Output
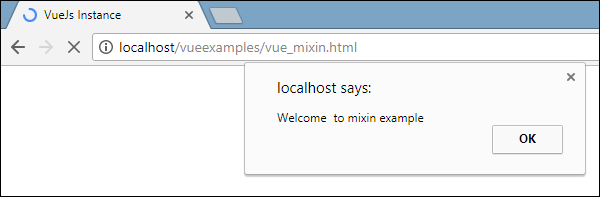
当mixin和组件包含重叠选项时,它们将合并,如以下示例所示。
<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"></div> <script type = "text/javascript"> var mixin = { created: function () { console.log('mixin called') } } new Vue({ mixins: [mixin], created: function () { console.log('component called') } }); </script> </body> </html>
现在,mixin和vue实例创建了相同的方法。这是我们在控制台中看到的输出。如图所示,vue和mixin的选项将合并。

如果我们碰巧在方法中具有相同的函数名称,则主vue实例将具有优先权。
Example

<html> <head> <title>VueJs Instance</title> <script type = "text/javascript" src = "js/vue.js"></script> </head> <body> <div id = "databinding"></div> <script type = "text/javascript"> var mixin = { methods: { hellworld: function () { console.log('In HelloWorld'); }, samemethod: function () { console.log('Mixin:Same Method'); } } }; var vm = new Vue({ mixins: [mixin], methods: { start: function () { console.log('start method'); }, samemethod: function () { console.log('Main: same method'); } } }); vm.hellworld(); vm.start(); vm.samemethod(); </script> </body> </html>
我们将看到mixin具有method属性,其中定义了helloworld和samemethod函数。类似地,vue实例具有一个methods属性,其中再次定义了两个方法start和samemethod。
以下每个方法均被调用。
vm.hellworld(); //在HelloWorld中 vm.start(); //启动方法 vm.samemethod(); // Main:相同的方法
如上所示,我们调用了helloworld,start和samemethod函数。 mixin中也存在samemethod,但是优先级将赋予主实例,如以下控制台所示。
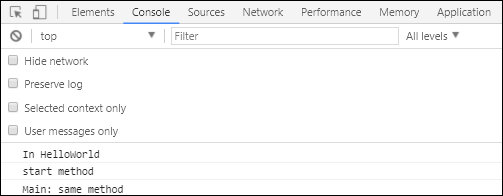