Angular 4 服务
在本章中,我们将讨论 Angular 4 中的服务。
我们可能会遇到需要在页面上到处使用一些代码的情况。它可以用于需要跨组件共享的数据连接等。服务帮助我们实现这一目标。通过服务,我们可以访问整个项目中其他组件的方法和属性。
要创建服务,我们需要使用命令行。相同的命令是:
C:\projectA4\Angular 4-app>ng g service myservice installing service create src\app\myservice.service.spec.ts create src\app\myservice.service.ts WARNING Service is generated but not provided, it must be provided to be used C:\projectA4\Angular 4-app>
app文件夹中创建的文件如下:
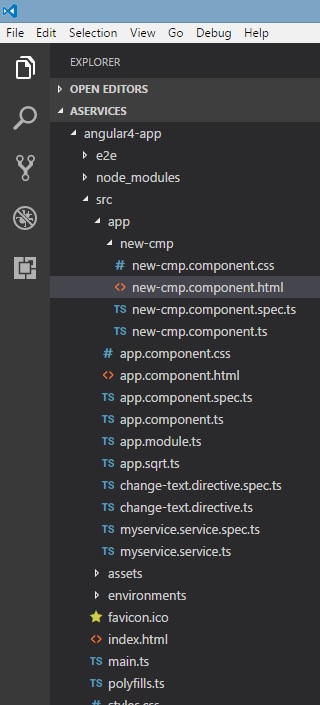
以下是在底部创建的文件 - myservice.service.specs.ts and 我的服务.service.ts .
我的服务.service.ts
import { Injectable } from '@angular/core'; @Injectable() export class MyserviceService { constructor() { } }
这里, Injectable 模块是从 @角/核心 .它包含 @可注入 方法和一个名为的类 我的服务 .我们将在这个类中创建我们的服务函数。
在创建新服务之前,我们需要将创建的服务包含在主父级中 app.module.ts .
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { RouterModule} from '@angular/router'; import { AppComponent } from './app.component'; import { MyserviceService } from './myservice.service'; import { NewCmpComponent } from './new-cmp/new-cmp.component'; import { ChangeTextDirective } from './change-text.directive'; import { SqrtPipe } from './app.sqrt'; @NgModule({ declarations: [ SqrtPipe, AppComponent, NewCmpComponent, ChangeTextDirective ], imports: [ BrowserModule, RouterModule.forRoot([ { path: 'new-cmp', component: NewCmpComponent } ]) ], providers: [MyserviceService], bootstrap: [AppComponent] }) export class AppModule { }
我们已经使用类名导入了服务,并且在提供程序中使用了相同的类。现在让我们切换回服务类并创建一个服务函数。
在服务类中,我们将创建一个显示今天日期的函数。我们可以在主父组件中使用相同的功能 app.component.ts 以及在新组件中 新 cmp.component.ts 我们在上一章中创建的。
现在让我们看看该函数在服务中的外观以及如何在组件中使用它。
import { Injectable } from '@angular/core'; @Injectable() export class MyserviceService { constructor() { } showTodayDate() { let ndate = new Date(); return ndate; } }
在上面的服务文件中,我们创建了一个函数 显示今日日期 .现在我们将返回新创建的 Date()。让我们看看如何在组件类中访问这个函数。
app.component.ts
import { Component } from '@angular/core'; import { MyserviceService } from './myservice.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 4 Project!'; todaydate; constructor(private myservice: MyserviceService) {} ngOnInit() { this.todaydate = this.myservice.showTodayDate(); } }
The ngOnInit 默认情况下,在创建的任何组件中都会调用函数。如上所示,从服务中获取日期。要获取服务的更多详细信息,我们需要首先将服务包含在组件中 ts file.
我们将日期显示在 .html 文件如下图:
{{todaydate}} <app-new-cmp></app-new-cmp> // 要从新组件类显示给用户的数据。
现在让我们看看如何在创建的新组件中使用该服务。
import { Component, OnInit } from '@angular/core'; import { MyserviceService } from './../myservice.service'; @Component({ selector: 'app-new-cmp', templateUrl: './new-cmp.component.html', styleUrls: ['./new-cmp.component.css'] }) export class NewCmpComponent implements OnInit { todaydate; newcomponent = "Entered in new component created"; constructor(private myservice: MyserviceService) {} ngOnInit() { this.todaydate = this.myservice.showTodayDate(); } }
在我们创建的新组件中,我们需要首先导入我们想要的服务并访问其方法和属性。请参阅突出显示的代码。今天的日期在组件html中显示如下:
<p> {{newcomponent}} </p> <p> Today's Date : {{todaydate}} </p>
新组件的选择器用于 app.component.html 文件。上述 html 文件中的内容将显示在浏览器中,如下所示:
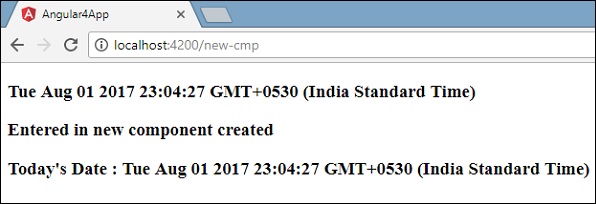
如果你在任何组件中更改服务的属性,那么在其他组件中也会发生相同的更改。现在让我们看看这是如何工作的。
我们将在服务中定义一个变量,并在父组件和新组件中使用它。我们将再次更改父组件中的属性,并查看新组件中是否更改了相同的属性。
In 我的服务.service.ts ,我们创建了一个属性并在其他父组件和新组件中使用了相同的属性。
import { Injectable } from '@angular/core'; @Injectable() export class MyserviceService { serviceproperty = "Service Created"; constructor() { } showTodayDate() { let ndate = new Date(); return ndate; } }
现在让我们使用 服务属性 其他组件中的变量。在 app.component.ts ,我们访问变量如下:
import { Component } from '@angular/core'; import { MyserviceService } from './myservice.service'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 4 Project!'; todaydate; componentproperty; constructor(private myservice: MyserviceService) {} ngOnInit() { this.todaydate = this.myservice.showTodayDate(); console.log(this.myservice.serviceproperty); this.myservice.serviceproperty = "component created"; // 值改变了。 this.componentproperty = this.myservice.serviceproperty; } }
我们现在将获取变量并在 console.log 上工作。在下一行中,我们将变量的值更改为“ 创建的组件 ”。我们将在 新 cmp.component.ts .
import { Component, OnInit } from '@angular/core'; import { MyserviceService } from './../myservice.service'; @Component({ selector: 'app-new-cmp', templateUrl: './new-cmp.component.html', styleUrls: ['./new-cmp.component.css'] }) export class NewCmpComponent implements OnInit { todaydate; newcomponentproperty; newcomponent = "Entered in newcomponent"; constructor(private myservice: MyserviceService) {} ngOnInit() { this.todaydate = this.myservice.showTodayDate(); this.newcomponentproperty = this.myservice.serviceproperty; } }
在上面的组件中,我们没有改变任何东西,而是直接将属性分配给组件属性。
现在,当你在浏览器中执行它时,服务属性将被更改,因为它的值在 app.component.ts 同样将显示为 新 cmp.component.ts .
在更改之前还要检查控制台中的值。
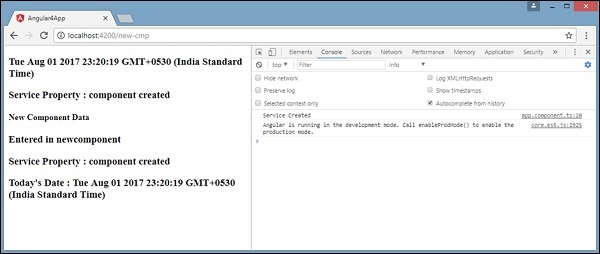