Angular 4 事件绑定
在本章中,我们将讨论事件绑定在 Angular 4 中是如何工作的。当用户以键盘移动、鼠标单击或鼠标悬停的形式与应用程序交互时,它会生成一个事件。需要处理这些事件以执行某种操作。这就是事件绑定发挥作用的地方。
让我们考虑一个例子来更好地理解这一点。
app.component.html
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1> Welcome to {{title}}. </h1> </div> <div> Months : <select> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable; then condition1 else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid</ng-template> <ng-template #condition2>Condition is invalid</ng-template> </div> <button (click)="myClickFunction($event)"> Click Me </button>
In the app.component.html 文件中,我们定义了一个按钮并使用 click 事件向它添加了一个函数。
以下是定义按钮并向其添加功能的语法。
(click)="myClickFunction($event)"
该函数定义在 .ts file: app.component.ts
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 4 Project!'; // 月份数组。 months = ["January", "Feburary", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = true; myClickFunction(event) { // 刚刚添加了console.log,它将在单击按钮时在浏览器中显示事件详细信息。 alert("Button is clicked"); console.log(event); } }
单击按钮后,控件将进入功能 我的点击函数 会出现一个对话框,显示 按钮被点击 如下图所示:
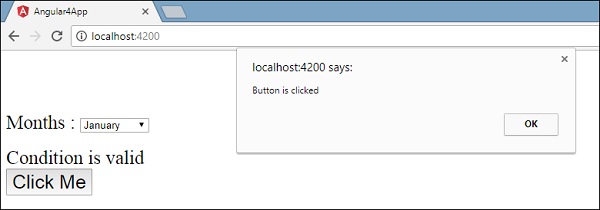
现在让我们将更改事件添加到下拉列表中。
以下代码行将帮助你将更改事件添加到下拉列表中:
<!--The content below is only a placeholder and can be replaced.--> <div style = "text-align:center"> <h1> Welcome to {{title}}. </h1> </div> <div> Months : <select (change) = "changemonths($event)"> <option *ngFor = "let i of months">{{i}}</option> </select> </div> <br/> <div> <span *ngIf = "isavailable; then condition1 else condition2"> Condition is valid. </span> <ng-template #condition1>Condition is valid</ng-template> <ng-template #condition2>Condition is invalid</ng-template> </div> <button (click) = "myClickFunction($event)">Click Me</button>
该函数声明在 app.component.ts file:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 4 Project!'; // 月份数组。 months = ["January", "Feburary", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = true; myClickFunction(event) { alert("Button is clicked"); console.log(event); } changemonths(event) { console.log("Changed month from the Dropdown"); console.log(event); } }
控制台消息“ 从下拉列表中更改月份 ”与事件一起显示在控制台中。

让我们在其中添加一条警报消息 app.component.ts 当下拉列表中的值发生更改时,如下所示:
import { Component } from '@angular/core'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Angular 4 Project!'; // 月份数组。 months = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"]; isavailable = true; myClickFunction(event) { // 刚刚添加了console.log,它将在浏览器中显示事件详细信息 on click of the button. alert("Button is clicked"); console.log(event); } changemonths(event) { alert("Changed month from the Dropdown"); } }
更改下拉列表中的值时,将出现一个对话框并显示以下消息 - “ 从下拉列表中更改月份 ”.
