Angular 2 表单
Angular 2 还可以设计可以使用双向绑定的表单,使用 ngModel 指示。让我们看看我们如何实现这一目标。
步骤 1 : 创建一个模型,它是一个产品模型。创建一个名为 产品.ts file.
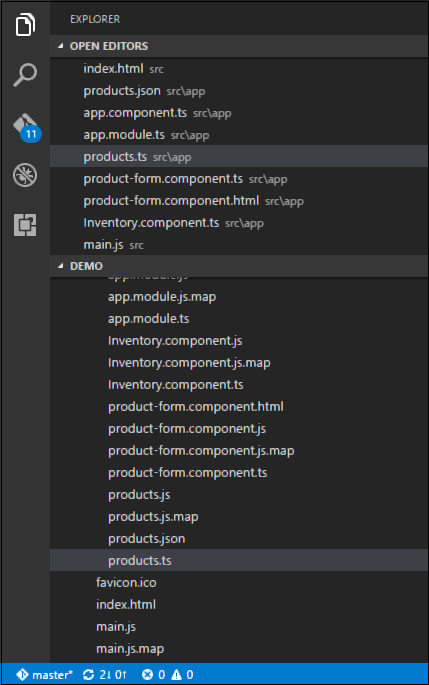
步骤 2 : 将以下代码放入文件中。
export class Product { constructor ( public productid: number, public productname: string ) { } }
这是一个简单的类,它有 2 个属性,productid 和 productname。
步骤 3 :创建产品表单组件product-form.component.ts组件,添加如下代码:
import { Component } from '@angular/core'; import { Product } from './products'; @Component ({ selector: 'product-form', templateUrl: './product-form.component.html' }) export class ProductFormComponent { model = new Product(1,'ProductA'); }
关于上述程序,需要注意以下几点。
-
创建 Product 类的对象并将值添加到 productid 和 productname。
-
使用 templateUrl 指定将呈现组件的 product-form.component.html 的位置。
步骤 4 : 创建实际表格。创建一个名为 product-form.component.html 的文件并放置以下代码。
<div class = "container"> <h1>Product Form</h1> <form> <div class = "form-group"> <label for = "productid">ID</label> <input type = "text" class = "form-control" id = "productid" required [(ngModel)] = "model.productid" name = "id"> </div> <div class = "form-group"> <label for = "name">Name</label> <input type = "text" class = "form-control" id = "name" [(ngModel)] = "model.productname" name = "name"> </div> </form> </div>
关于上述程序,需要注意以下几点。
-
The ngModel 指令用于将产品的对象绑定到表单上的单独元素。
步骤 5 : 将以下代码放在app.component.ts文件中。
import { Component } from '@angular/core'; @Component ({ selector: 'my-app', template: '<product-form></product-form>' }) export class AppComponent { }
步骤 6 : 将以下代码放在app.module.ts文件中
import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { AppComponent } from './app.component'; import { FormsModule } from '@angular/forms'; import { ProductFormComponent } from './product-form.component'; @NgModule ({ imports: [ BrowserModule,FormsModule], declarations: [ AppComponent,ProductFormComponent], bootstrap: [ AppComponent ] }) export class AppModule { }
步骤 7 : 保存所有代码并使用 npm 运行应用程序。转到你的浏览器,你将看到以下输出。
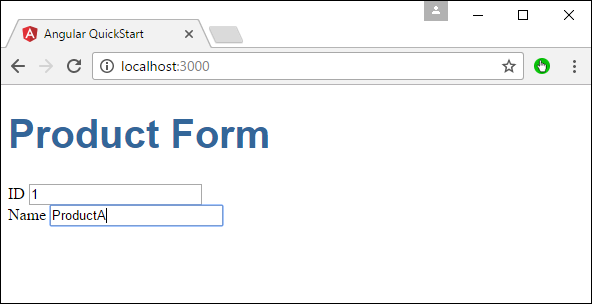